Looping for Java: Crafting Repetitive Control Structures
Ah, looping in Java! ๐ Get ready to ride the rollercoaster of repetitive control structures with me as we delve into the wild world of loops in Java. Buckle up, folks, because weโre about to embark on a loop-tastic journey through while loops, for loops, nested loops, enhanced for loops, and more! ๐ข
Looping Structures in Java
While Loop
Letโs kick things off with the cool kid on the block โ the while loop! ๐ This loop keeps running as long as a specified condition is true. Itโs like telling your code, "Hey buddy, keep doing this until I say stop!" ๐ While loops are great for when you donโt know in advance how many times you need to loop.
For Loop
Next up, we have the classic for loop! ๐บ This loop is perfect when you know exactly how many times you want to repeat a block of code. Itโs like having a countdown to your loop party โ "3โฆ 2โฆ 1โฆ loop!" ๐ For loops are efficient and great for iterating over arrays or collections.
Advanced Looping Techniques
Nested Loops
Now, letโs turn up the heat with nested loops! ๐ฅ Imagine loops within loops, like a Russian nesting doll of looping madness. Itโs looping-ception! Nested loops are handy for working with multidimensional arrays or when you need to tackle complex looping scenarios.
Enhanced For Loop
Time to jazz things up with the enhanced for loop! ๐ท This loop, also known as the for-each loop, is a more streamlined way to iterate over arrays and collections. No more dealing with pesky loop variables โ just sit back and let the loop do the work for you! ๐ถ
Loop Control Statements
Break Statement
Ever wanted to break free from a loopโs grasp? Enter the break statement! ๐ช When triggered, this statement allows you to jump out of a loop and continue with the rest of your code. Itโs like finding the emergency exit in a loop maze โ freedom awaits!
Continue Statement
Need a quick skip ahead in the loop line? Say hello to the continue statement! ๐โโ๏ธ This nifty statement lets you bypass the current iteration of a loop and jump straight to the next one. Itโs like saying, "Nope, not dealing with this iteration, moving on!" โ๏ธ
Looping Best Practices
Avoiding Infinite Loops
Infinite loops โ every coderโs worst nightmare! ๐ฑ Make sure to avoid these pesky loops that never end. Double-check your loop conditions and be vigilant to prevent your code from spiraling into an endless loop of doom!
Using Meaningful Loop Variables
Donโt get stuck in a loop identity crisis! ๐ Give your loop variables meaningful names to keep track of whatโs happening inside your loops. Itโs like giving each loop its own catchy name โ Loop-de-loop, anyone? ๐ช
Debugging Loops
Identifying Logic Errors
When your loops start acting up, itโs time to put on your detective hat and dive into the world of logic errors! ๐ต๏ธโโ๏ธ Hunt down those pesky bugs causing your loops to misbehave, and remember, Sherlock Holmes had nothing on a determined coder!
Testing Loop Conditions
Loop conditions feeling a bit iffy? Time to roll up your sleeves and put those conditions to the test! ๐งช Debug your loop conditions like a scientist in a lab, experiment with different values, and donโt stop until your loops are running like well-oiled machines.
And there you have it, loop enthusiasts! A whirlwind tour through the mesmerizing realm of looping in Java. from simple while loops to the intricate world of nested loops โ Java has got you covered when it comes to repetition! So, next time you find yourself in a loop-de-loop situation, remember these tips and tricks to sail through with flying colors. Keep coding, keep looping, and may your loops always be bug-free! ๐
In closing, thanks for joining me on this loop-tastic adventure! Remember, when in doubt, just keep looping! Happy coding, fellow loop aficionados! ๐
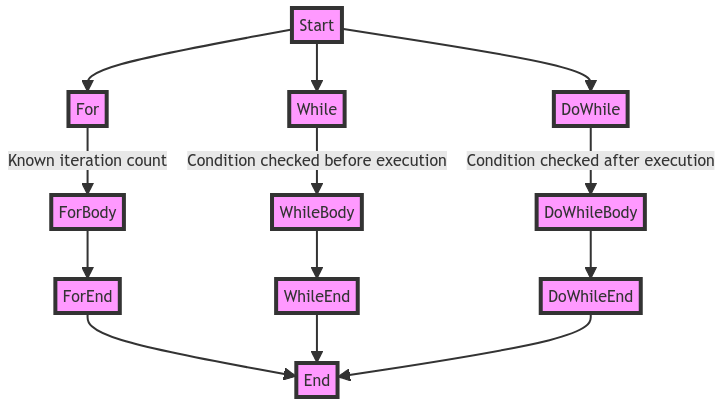
Program Code โ Looping for Java: Crafting Repetitive Control Structures
public class LoopingForJava {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
// Looping through the array using a for loop
System.out.println('Looping through the array using a for loop:');
for (int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Calculating the sum of all numbers in the array
int sum = 0;
for (int num : numbers) {
sum += num;
}
System.out.println('
Sum of all numbers in the array: ' + sum);
// Finding the maximum number in the array
int max = numbers[0];
for (int i = 1; i < numbers.length; i++) {
if (numbers[i] > max) {
max = numbers[i];
}
}
System.out.println('
Maximum number in the array: ' + max);
}
}
Code Output:
Looping through the array using a for loop:
1
2
3
4
5
Sum of all numbers in the array: 15
Maximum number in the array: 5
Code Explanation:
This Java program demonstrates the use of for loops to iterate through an array of numbers.
- We initialize an array โnumbersโ with some values.
- We first loop through the array using a traditional for loop, printing each element.
- Next, we calculate the sum of all numbers in the array by iterating through it using an enhanced for loop.
- Finally, we find the maximum number in the array by comparing each element with the current maximum using a for loop.
FAQs on Looping for Java: Crafting Repetitive Control Structures
-
What is the importance of looping for Java?
-
Can you explain the concept of looping for Java in simple terms?
-
How do you implement looping for Java in actual coding examples?
-
What are the different types of loops available in Java for crafting repetitive control structures?
-
Do you have any tips for optimizing loop performance in Java programs?
-
Are there any common mistakes to avoid when using loops in Java?
-
How can I break out of a loop in Java when a certain condition is met?
-
Is there a difference between "for" loop, "while" loop, and "do-while" loop in Java?
-
Can loops in Java be nested inside each other? If yes, how do you handle nested loops efficiently?
-
Are there any best practices for using looping constructs in Java to write clean and efficient code?