Python is a very popular programming language and it is widely used by students, programmers, and researchers. The Python programming language provides a large number of functionalities and the mix-iterator is one of those features.
When it comes to programming we use different types of iterators in Python to perform a specific task. Iterators are the most efficient way to manipulate data in Python and it is the most popular feature of Python. It is basically the collection of functions that works as a loop. It will create a new element of a sequence every time it is called.
Mix-iterators is a feature that allows the user to combine several iterators into one single iterator. It is an advanced feature that is used when the data needs to be processed in different ways. It is very helpful to save space and time as compared to creating multiple iterators.
In this article, we will discuss the five best tips to use the mixing iterators in Python.
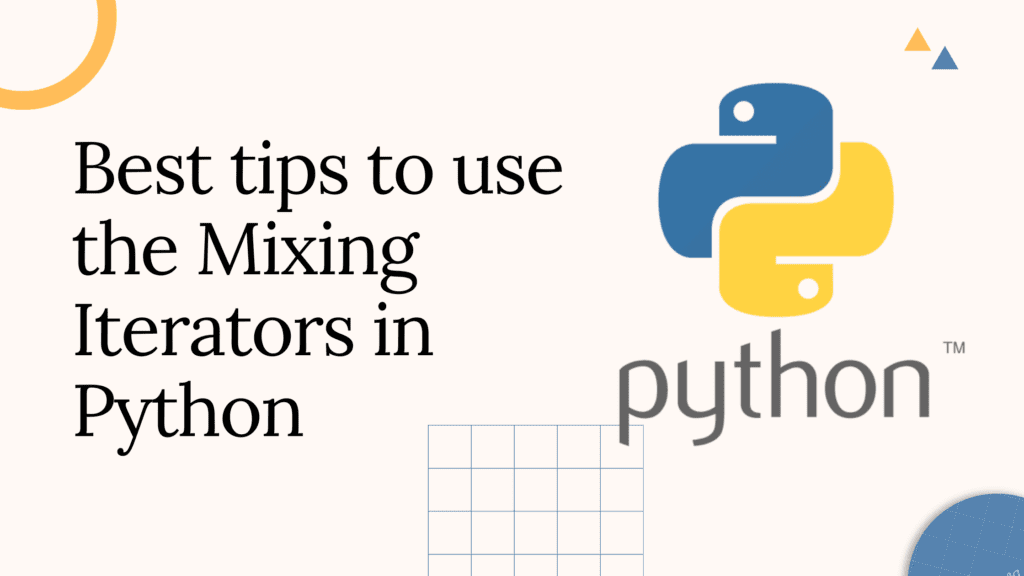
Using the yield statement Python mixing iterator
Yield is one of the most important tools that is used in the Python programming language. It is a feature that will allow the users to create an iterator.
The yield statement is also known as the “yield from”. This statement is used to create an iterator from a function or a generator. When a yield statement is used, it will return all the values as a list or a dictionary.
def fibo():
a, b = 1, 1
while True:
yield a
a, b = b, a+b
def alter():
n = 1
while True:
yield n
if n < 0:
n -= 1
else:
n += 1
n *= -1
Sometimes we want to mix the different types of iterators to perform a certain task.
For example, we want to combine the range and enumerate iterators to generate the prime numbers between two values. Here is the code.
primes = []
for i in range(2, 100):
if not i.is_prime():
primes.append(i)
print(primes)
In Python, the iterators are defined as the objects that are used to define the sequence. So, the mixing iterator is a kind of iterator and it is also a generator. The main purpose of a generator is to produce the next item in a sequence.
A mixing iterator produces items of different types, so if you are looking for the different kinds of iterators then you should check the Python documentation.
A mixing iterator is a generator that produces a sequence of values of a different type. Let me explain the concept by taking an example.
Suppose you have a list of integers. You don’t want the whole list to print in a single line and instead you want to print the numbers in a particular format. For example, if you want to print the list in ascending order, you can use the following code.
l = [1, 2, 3, 4, 5, 6]
for i in l:
print(f'{i}')
In this example, the loop is executed once and the output will be the list in ascending order.
But, if you want to display the list in descending order, then you will need a different approach. So, if you are looking for a solution then you can use the mixing iterator.
l = [1, 2, 3, 4, 5, 6]
print('\n')
for i in range(len(l)):
j = random.randint(0, len(l)-1)
print(f'{l[i]} - {l[j]}')
This example shows that the python iterators can be used to mix elements of different types.